Page Contents
Quick summary:
Writing clean and precise code is indeed a difficult task for any developer. To keep up with the client’s growing demand, you may have to modify existing modules or add more lines of code which might result in performance issues. React.js is a popular JavaScript library that makes it easier to write clean and maintainable code for your project. However, achieving the perfect balance depends on adopting the right techniques. This blog helps you with the top 10 React.js best practices and tips to follow while React.js Application development.
Introduction
Imagine your client demands to build a highly interactive UI with multiple components. What choice will you make first? You may decide to write code for each of those interactive components separately and connect them to the underlying BLL (business logic layer). Well, React may help you save both your time and effort.
React.js is a popular JavaScript library employed for building interactive UIs (user interfaces), and following best practices can help you ensure your React app is quick, efficient, and reliable. Here are the top 10 React.js best practices and tips to follow.
-
Proper structure of folders and files
Beginning your React app project well makes the rest easier. Remember the adage “a job well begun is half done”? The same applies here.
Use the following npm command to create a new basic structure.
create-react-app
Your project folder and structure would look like this.
Make sure you start the project appropriately; you won’t have to put in much effort to finish it. Because ReactJS is a component-based JavaScript framework, it is best to categorize files according to their kind. Hooks go in the Hooks folder, components go in the Components folder, context goes in the Context folder, and so on.
-
Use functional components wherever possible
Many individuals, particularly beginners, get stuck on the question of whether to use a class component or a functional component. The solution is functional components since they are easier to comprehend, test, and debug than class-based components. Furthermore, these might help to improve the performance of your application.
Here is the sample code for the Functional component
Here is an example of class component code.
Benefits of using Functional components:
- Simple to use, test, and debug.
- Written in simple JS, with no use of lifecycle or state hooks.
- Fewer lines of code
- No need to use the ‘this’ keyword explicitly.
Class components are preferred when you have the need for managing state.
-
Use DevTools (the React Developer Tools) browser extension
Installing the React-specific DevTools extension in your browser allows you to inspect and debug the components of your React.js application to learn about the current actions.
When you install this extension, you will have access to two crucial features. The first is a component tree view, while the second represents their current state and properties.
Some crucial advantages of using React Devtools (extension) are
- Minimal code and flawless testing
- Fine flexibility for smaller components
- Stateless components to focus on UI rather than behavior
- Use React.memo higher-order component
This component helps you to enhance your application’s speed by only re-rendering a component if its properties have changed. What makes this so interesting is that it aids in the elimination of mutating or repetitive logic in other components.
When can we use React.memo?
Suppose you have a need to render the parent component with its values, the child components will be forced to re-render with the same values. So, to deal with this situation, React.memo can be used.
-
Use React.useMemo() hook
Using higher-order components like this can help you enhance the speed of your functional components. It allows you to cache the outcome of computation between re-renders.
React.useMemo() hook can be used in three steps – import, compute and render.
Step- 1: To begin with, import the hook from the component-based React.js library.
Syntax:
import { useMemo } from “react”;
Step- 2: Next, proceed with computing React.useMemo() hook.
Syntax:
const memoValue = useMemo(() => {/* function */}, [/* Dependencies */]);
Step – 3: Render the hook for desired output.
Syntax:
<div className=”App”>{memoValue}</div>
-
Optimize child components using the React.useCallback() hook
With this functional hook, you can improve the performance of functions that are passed as props to child components.
Unless there is any change in function, React.useCallback() avoids the re-rendering of a child component.
Also Read: 5 Mobile App development trends to look in 2023
-
Employing the useEffect hook
Using this useEffect() hook, you can perform and eliminate side effects in your class-based components. Some examples are – making API calls, setting up subscriptions, updating DOM, and so on.
In what situations can usEffect be useful?
When you are unable to access your database or the logging services have reached their capacity, the usEffect might save you time.
-
Use the React Router library
You may handle routing in your React application with this standard Routing module. It provides for smooth navigation between various component views, allows for browser URL adjustment, and maintains the front end in sync with the URL.
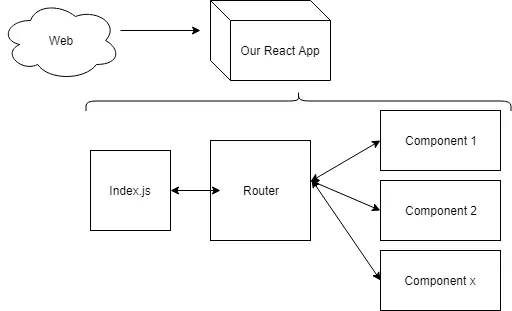
-
Apply ES6 Spread Function
It is recommended to use ES6 methods for sending object attributes as they are simple and effective. When “{…props}” is used in the code, the object’s properties are instantly accessible. Look at the example shown below.
let pList = { className: “selected-props “,
id: “selected”,
content: “Hello, let’s take a look at the selected props”};
let SmallDiv = props => <div {… props} />;
let mainDiv = < SmallDiv props={pList} />;
The spread function eliminates the need for ternary operators and enables the usage of HTML tag attributes rather than the entire content. However, it is suggested not to use spread functions with nested tags, and array characteristics.
-
Use React Testing Library to write Tests
Writing code and creating modules do not guarantee the success of any application. Rather, it is determined by how thoroughly you test the app and resolve any bugs that arise.
With the best use of React testing library, you may develop easy and manageable test cases for your React.js app components.
Also read: Benefits of FinTech applications and technology
So that brings us to the end of this article. If you follow these design principles, you will be able to create a flawless and long-lasting application for your business.
Conclusion
React.JS turns out to be the best platform for UI development. The main objective of ReactJS is to deliver simple, quick, and incredibly scalable applications. Moreover, its code reusability feature helps you with minimized code and high performance for your business application.
When you develop an application using React, it is necessary to pay close attention to the modifications, or else the code could become cluttered and difficult to maintain. Despite being the best choice for front-end development, it is important to follow certain practices to get the most out of it.
In this article, we discussed the Top 10 React.js best practices and suggestions to follow in 2023 to help you make the most out of your ReactJS application.